SpringBoot集成Thymeleaf
前面章节我们介绍了SpringBoot集成jsp和Freemarker以及它们的具体应用。而在这些前端模板引擎中,SpringBoot首推使用Thymeleaf。这是因为Thymeleaf对SpringMVC提供了完美的支持。
Thymeleaf简介
Thymeleaf同样是一个Java类库,能够处理HTML/HTML5、XML、JavaScript、CSS,甚⾄纯⽂本。通常可以用作MVC中的View层,它可以完全替代JSP。
Thymeleaf的特性
- Thymeleaf不仅可以作为模板存在,同时也支持HTML原型。通过在HTML标签里增加额外的属性来达到模板+数据的展示方式。浏览器解释HTML时会忽略未定义的标签属性,所以可直接通过浏览器打开;当有数据返回到页面时,Thymeleaf标签会动态地替换掉静态内容,使页面动态显示。
- Thymeleaf开箱即用的特性。它支持标准方言和Spring方言,可以直接套用模板实现JSTL、OGNL表达式效果,避免重复套模板、改JSTL、改标签的困扰。同时开发人员也可以扩展和创建自定义的方言。
- Thymeleaf提供Spring标准方言和一个与SpringMVC完美集成的可选模块,可以快速地实现表单绑定、属性编辑器、国际化等功能。
与其他模板引擎相比,Thymeleaf不会破坏文档结构。对比Freemarker可以看出效果:
FreeMarker: <p>${message}</p> Thymeleaf: <p th:text="${message}">Hello World!</p>
注意,由于Thymeleaf使用了XML DOM解析器,因此它并不适合于处理大规模的XML文件。
实例演示
SpringBoot中创建项目并集成Thymeleaf。创建过程中勾选对应集成框架。
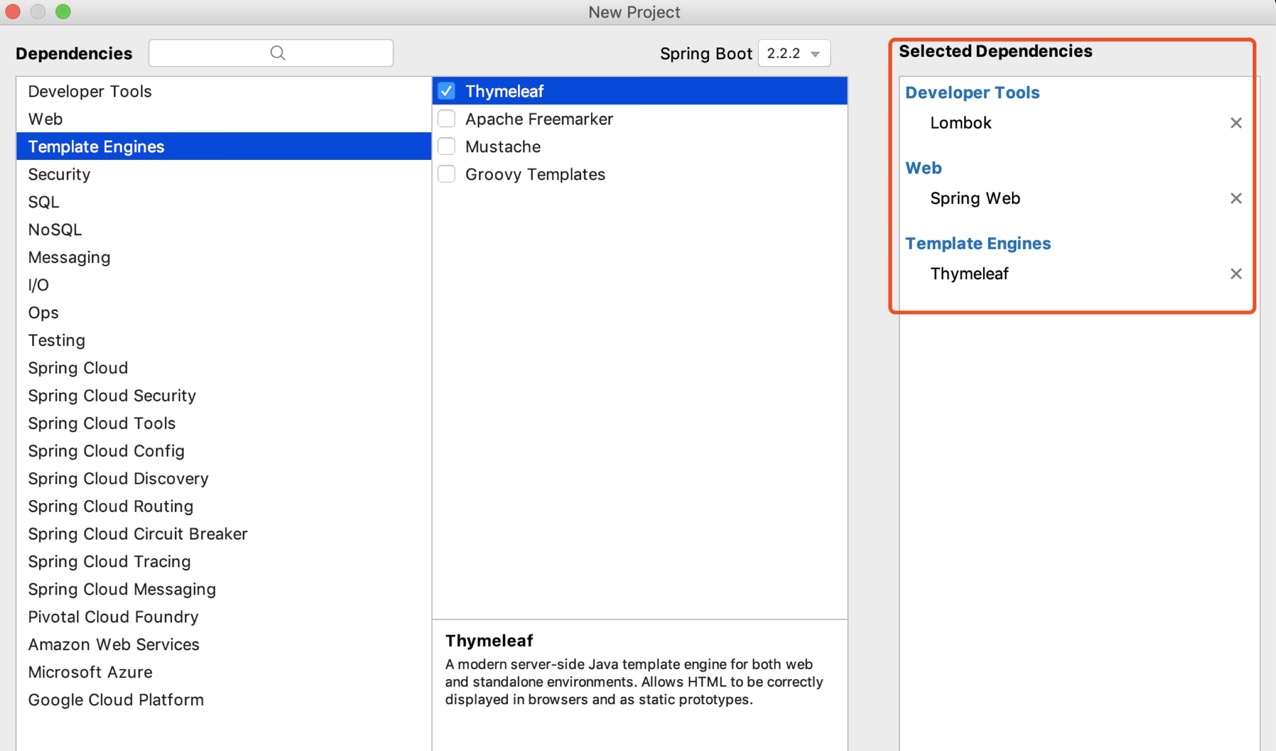
项目创建之后,pom中对应的核心依赖如下:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> </dependency>
实体类Student:
@Data public class Student { private String idNo; private String name; }
Controller对应实现:
@Controller public class StudentController { @GetMapping("/") public String getStudents(Model model){ List<Student> list = new ArrayList<>(); Student s1 = new Student(); s1.setIdNo("N01"); s1.setName("Tom"); list.add(s1); Student s2 = new Student(); s2.setIdNo("N02"); s2.setName("David"); list.add(s2); model.addAttribute("students",list); model.addAttribute("hello","Hello Thymeleaf!"); return "student"; } }
在Controller中实现了两个参数的返回一个为字符串,一个为Student的列表。
对应templates下的页面为student.html。Thymeleaf默认使用html作为后缀。
student.html页面展示信息如下:
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"/> <title>Hello Thymeleaf</title> </head> <body> <h1 th:text="${hello}">Hello World</h1> <div th:each="student : ${students}"> <p th:text="${student.name} + ':' + ${student.idNo}"></p> </div> </body> </html>
其中第一个为直接展示字符串,第二个为遍历列表并展示其中的属性值。
访问对应请求http://localhost:8080/,即可返内容展示。
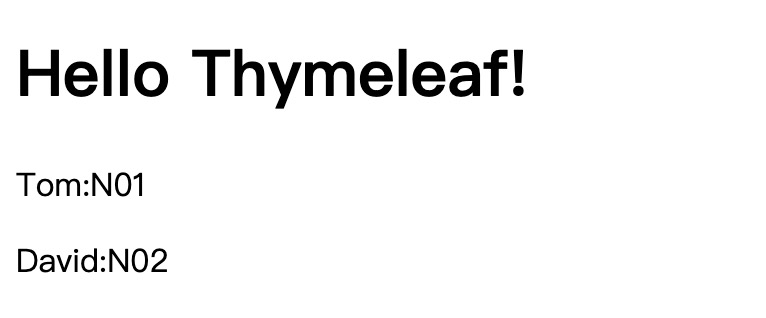
注意事项
如果是在开发环境中,最好在application.properties中添加配置:
spring.thymeleaf.cache=false
关闭Thymeleaf的缓存(默认为true),避免因缓存导致修改需重启才能生效,生产环境可采用默认值。
使用Thymeleaf的页面必须在HTML标签中作如下声明,表示使用Thymeleaf语法:
<html xmlns:th="http://www.thymeleaf.org">
SpringBoot中相关配置
SpringBoot中提供了大量关于Thymeleaf的配置项目:
# 开启模板缓存(默认值:true) spring.thymeleaf.cache=true # 检查模板是否存在 spring.thymeleaf.check-template=true # 检查模板位置是否正确(默认值:true) spring.thymeleaf.check-template-location=true # Content-Type的值(默认值:text/html) spring.thymeleaf.content-type=text/html # 开启MVC Thymeleaf视图解析(默认值:true) spring.thymeleaf.enabled=true # 模板编码 spring.thymeleaf.encoding=UTF-8 # 排除视图名称列表,用逗号分隔 spring.thymeleaf.excluded-view-names= # 模板模式,设置为HTML5会严格校验,不符合规则将报错 spring.thymeleaf.mode=HTML5 # 视图名称前缀(默认值:classpath:/templates/) spring.thymeleaf.prefix=classpath:/templates/ # 视图名称后缀(默认值:.html) spring.thymeleaf.suffix=.html # 可解析的视图名称列表,用逗号分隔 spring.thymeleaf.view-names=
关于SpringBoot集成Thymeleaf的操作已经完成,非常简单。
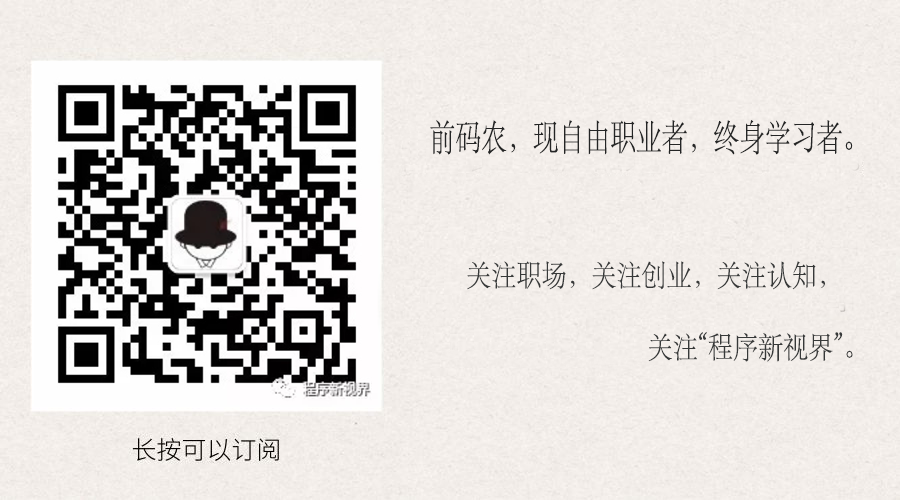
关注公众号:程序新视界,一个让你软实力、硬技术同步提升的平台
除非注明,否则均为程序新视界原创文章,转载必须以链接形式标明本文链接
本文链接:https://www.choupangxia.com/2020/01/14/springboot2-x-springboot-thymeleaf/