springboot Junit5 单元测试
Junit5简介
JUnit 5跟以前的JUnit版本不一样,它由几大不同的模块组成,这些模块分别来自三个不同的子项目。
JUnit 5 = JUnit Platform + JUnit Jupiter + JUnit Vintage
核心注解
- @BeforeAll 类似于JUnit 4的@BeforeAll,表示使用了该注解的方法应该在当前类中所有使用了@Test、@RepeatedTest、@ParameterizedTest或者
- @TestFactory注解的方法之前执行,必须为static。
- @BeforeEach 类似于JUnit 4的@Before,表示使用了该注解的方法应该在当前类中每一个使用了@Test、@RepeatedTest、@ParameterizedTest或者@TestFactory注解的方法之前执行。
- @Test 表示该方法是一个测试方法。
- @DisplayName 为测试类或测试方法声明一个自定义的显示名称。
- @AfterEach 类似于JUnit 4的@After,表示使用了该注解的方法应该在当前类中每一个使用了@Test、@RepeatedTest、@ParameterizedTest或者@TestFactory注解的方法之后执行。
- @AfterAll 类似于JUnit 4的@AfterClass,表示使用了该注解的方法应该在当前类中所有使用了@Test、@RepeatedTest、@ParameterizedTest或者@TestFactory注解的方法之后执行,必须为static。
- @Disable 用于禁用一个测试类或测试方法,类似于JUnit 4的@Ignore。
- @ExtendWith 用于注册自定义扩展。
添加依赖
spring-boot-starter-test默认使用junit4,需要手动添加junit5依赖。可通过两种方式来添加:
<!-- Junit 5 --> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter</artifactId> <version>5.5.2</version> <scope>test</scope> </dependency>
或通过如下方式:
<!-- Junit 5 --> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-params</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <scope>test</scope> </dependency>
如果必要则可将spring-boot-starter-test中的junit-vintage-engine排除。
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency>
代码示例
package com.secbro2.learn.service; import com.secbro2.learn.model.Order; import org.junit.jupiter.api.Test; import org.springframework.boot.test.context.SpringBootTest; import javax.annotation.Resource; //@ExtendWith(SpringExtension.class) @SpringBootTest public class OrderServiceTest { @Resource private OrderService orderService; @Test public void testInsert() { Order order = new Order(); order.setOrderNo("A001"); order.setUserId(100); orderService.insert(order); } }
其中需要注意的是如果是spring boot 2.1.x之后版本只使用@SpringBootTest即可,如果是之前版本,还需要添加@ExtendWith(SpringExtension.class)注解,否则无法生效。
相关文章:
《SPRING BOOT JUNIT5单元测试@EXTENDWITH无法工作》
《SPRING BOOT JUNIT5单元测试注入BEAN抛NULLPOINTEREXCEPTION空指针异常》
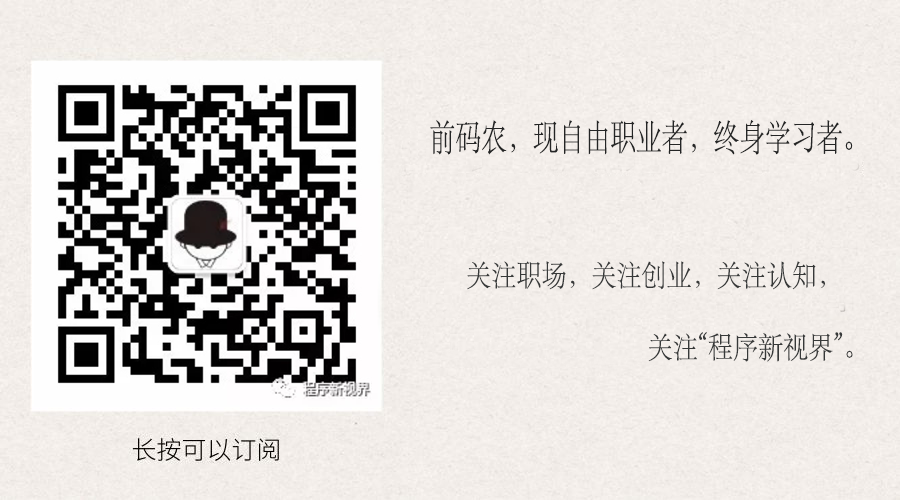
关注公众号:程序新视界,一个让你软实力、硬技术同步提升的平台
除非注明,否则均为程序新视界原创文章,转载必须以链接形式标明本文链接
本文链接:http://www.choupangxia.com/2019/11/13/springboot-junit5-demo/